If there are any devs lurking around here, I could use some help
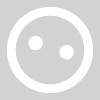
I'm trying to figure out if I'm dealing with faulty hardware, bad code (mine), bad firmware/drivers, or something else. I have a clubsport wheel base v 2.5 with a wheel and CSL Elite Pedal set. They were acquired around 2019 sometime, I think.
I have been unable to get the pedal set to work using the cable that connects the pedal set box to the wheelbase. I have been able to get the pedal set working independently via the USB connector. I have been playing around a little with Asseto Corsa, but have yet to get FF working.
I have created a super basic forms app that just applies a CCW constant force at the wheel axis. It works with a Logitech G29 just fine. I cannot get the Fanatec Wheelbase to respond to any FF at all. I have a much larger more comprehensive application that allows device selection and all of the DirectInput parameters can be changed and are linked to form controls and they all work awesome with a G29, again, nothing with the Fanatec.
Full disclosure, I'm seeing cracked plastic and suspect this has been dropped at least once. Still, this stuff is built like a tank...consistent with traditional German engineering reputation. :) I'm not able to provide any code. Seems the forum attachment filter prevents that. Although I will probably ask a question on Stackoverflow or stackecxhange. I'll try to paste it into the content of this post.
using SharpDX.DirectInput;
namespace FanatecFormFF {
public partial class Form1 : Form
{
readonly DirectInput directInput;
readonly Joystick wheel;
Effect effect;
int[] _actuatorObjectIds;
int[] dirVector;
public Form1()
{
InitializeComponent();
var directInput = new DirectInput();
// Initialize DirectInput
var actuatorsLst = new List<int>(1);
// Find and initialize the Fanatec wheel
var devices = directInput.GetDevices(DeviceType.Driving, DeviceEnumerationFlags.AttachedOnly);
if (devices.Count == 0)
{
Console.WriteLine("No Fanatec wheel found.");
return;
}
wheel = new Joystick(directInput, devices[0].InstanceGuid);
foreach (var obj in wheel.GetObjects())
{
if (obj.ObjectId.Flags.HasFlag(DeviceObjectTypeFlags.ForceFeedbackActuator))
actuatorsLst.Add((int)obj.ObjectId);
//if (!obj.ObjectId.Flags.HasFlag(DeviceObjectTypeFlags.NoData))
// dataGenerators.Add(obj);
}
_actuatorObjectIds = [.. actuatorsLst];
dirVector = Enumerable.Repeat(1, _actuatorObjectIds.Length).ToArray();
wheel.Properties.BufferSize = 128;
}
private void Form1_Shown(object sender, EventArgs e)
{
wheel.SetCooperativeLevel(Handle, CooperativeLevel.Exclusive | CooperativeLevel.Foreground);
wheel.Acquire();
}
private void button1_Click(object sender, EventArgs e)
{
// Set up the force feedback effect
var constantForce = new ConstantForce
{
Magnitude = 5000 // Set the force magnitude (range is typically -10,000 to 10,000)
};
var effectParameters = new EffectParameters
{
Flags = EffectFlags.Cartesian | EffectFlags.ObjectIds,
Duration = int.MaxValue,
SamplePeriod = 0,
Gain = 10000,
TriggerButton = -1,
TriggerRepeatInterval = int.MaxValue,
Axes = _actuatorObjectIds,
Directions = dirVector,
StartDelay = 0,
Envelope = null,
Parameters = constantForce
};
//// Create the effect
//var effectInfo = new EffectInfo
//{
// Guid = EffectGuid.ConstantForce
//};
effect = new Effect(wheel, EffectGuid.ConstantForce, effectParameters);
// Start the effect
effect.Start(1, EffectPlayFlags.NoDownload);
}
private void button2_Click(object sender, EventArgs e)
{
// Stop the effect
effect.Stop();
}
}
}